This is a guide for the beginner or intermediate web developer who wants to know what frontend web frameworks to use.
Part 1: How and why the web was created.
Part 2: How JavaScript became what it is today. Read to understand the complex landscape of JavaScript frameworks and tools.
Part 3: CSS, pre-processors and UI frameworks.
Part 4: A guide for choosing a Frontend framework.
TL;DR
Choosing frontend frameworks
Overall winner: React and Next.js + TailwindCSS
React is a JavaScript frontend framework for making highly interactive UIs.
TailwindCSS is a utility-first CSS framework for rapid UI development.
Use Next.js (a React meta-framework) if you need server-side rendering (SSR), e.g., for Search-Engine Optimization (SEO).
For websites that needs any sort of interactive UI (which includes most modern websites and apps), React is a good choice. It has been the most used javascript frontend web framework for at least five years and the community is huge.
Alternative for very simple websites: jQuery / Alpine.js + Bootstrap
jQuery is a JavaScript library designed to simplify HTML DOM tree traversal and manipulation, as well as event handling, CSS animation, and Ajax. It has an easy-to-use API that works across a multitude of browsers. Installing jQuery is easy, just add a script tag with a CDN link in your HTML document.
Alpine.js is a minimal tool for composing behavior and interactive UIs directly in your markup. Think of it like jQuery for the modern web. Installing it is as easy as jQuery, just pop in a script tag and get going.
Bootstrap is a CSS toolkit with grids, ready-made components, icons, and more. They have a CDN version that can be included directly into the HTML document.
Components of a web app
To recap from previous parts, the components of a web app are:
Frontend, which lives in the browser on the device that loads the website/app. The frontend creates the UI that a user interacts with.
Backend, which lives on a server somewhere, usually in the cloud. The frontend makes requests to the backend to provide data or perform tasks. Common backend tasks are authentication and accessing data from a database. If your app/website only uses static data and you don't need authentication or other backend features, then you can run your app/website without a backend.
Third-party services, that your app uses to add functionality or data from a third party. E.g., utilizing Google Maps in your app, or getting data from a weather service.
Frontend uses HTML, CSS and JavaScript to create a website with content and interactivity. Modern web development uses various JavaScript web frameworks and tools to make it easier to develop high quality frontends for websites and web apps.
If you need backend features, there are several options. The easiest option is to use a server-less backend service such as Firebase to run and manage a backend for you. Firebase have services for databases, authentication, notifications, and more. Another option is to rent a server and manage the backend yourself. Usually you rent a virtual machine at AWS, Azure, Google Cloud, Linode, Heroku, or similar. Then you code your backend yourself with a framework like Django (python) or Express (node.js). A third option is to run a server yourself, but it is more hassle than its worth for most people.
In the rest of this article we will only discuss frontend web frameworks.
Frontend Web Frameworks
The list of Frontend frameworks is seemingly endless, and there seems to always be a new framework around the corner. It is a large, opinionated and complex landscape that is hard to navigate if you are new to web development.
Over the years, frontend web frameworks and the tools they depend on have become increasingly more complex, making it easier to make great apps and websites, but making it harder to understand how the web actually works. I described the evolution of JavaScript tools and frontend frameworks in part 2 of this series, and I recommend reading it if you don’t know what node.js, bundlers, or a meta framework is:
Frontend web frameworks can be roughly categorized in the following camps:
JavaScript libraries and progressive frameworks that can be directly imported into your HTML file without a build step. Examples are:
jQuery
Alpine.js
Vue.js
Single-Page Applications (SPAs) frameworks, that require a build step (e.g., with node.js and webpack) and introduces concepts such as reactivity and components. Examples are:
Vue
React
Svelte
Angular
Meta/hybrid frameworks, that does the same as SPAs, but where data can be rendered on the server, aka. Server-Side Rendering (SSR), and gives even more structure of how to do things. These take on some of the backend responsibilities, but not all, and a full-scale backend framework is usually preferred for applications that are backend heavy. Examples are:
Next.js (React)
Remix (React)
Nuxt (Vue)
Angular Universal (Angular)
SvelteKit (Svelte)
Choosing a Frontend framework
First of all: There is no clear-cut answer. Even though they slightly differ in how they do things, all of these frameworks can create great apps. If you don’t know or care which framework or features you want, I recommend choosing one based on popularity/usage, community size, and enjoyment.
We will use these three metrics to find winning framework below. The data will be based on surveys, StackOverflow stats, and my own experience. The surveys in question are The State of JS 2021 Survey (2022 is not out yet) and the Stack Overflow Developer Survey (2022).
Meta/hybrid frameworks will not be considered here, as they are linked to the frontend framework of choice (Next.js or Remix for React, Nuxt for Vue, Angular Universal for Angular, SvelteKit for Svelte).
Usage numbers
According to both The State of JS 2021 and the Stack Overflow Developer Survey (2022), React is currently the most used frontend web framework, and has enjoyed that spot for many years. React came out in 2013, the first of these modern web frameworks.
In The State of JS survey, we have Angular, Vue.js, and Svelte in descending order after React. In this survey they did not consider jQuery as a framework, so it is not listed.
In the Stack Overflow Developer Survey, we can see that jQuery is number two behind React, and then Angular, Vue and Svelte. Even if jQuery is by many considered an old library, is still widely used in the web today, but this is mostly for websites with simple UIs (for which it is a good choice, IMO). Note that this comparison includes backend frameworks and runtimes as well.
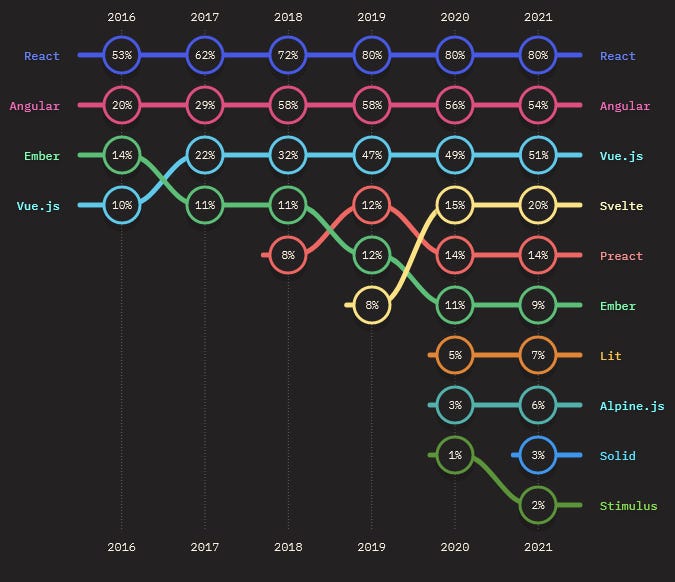
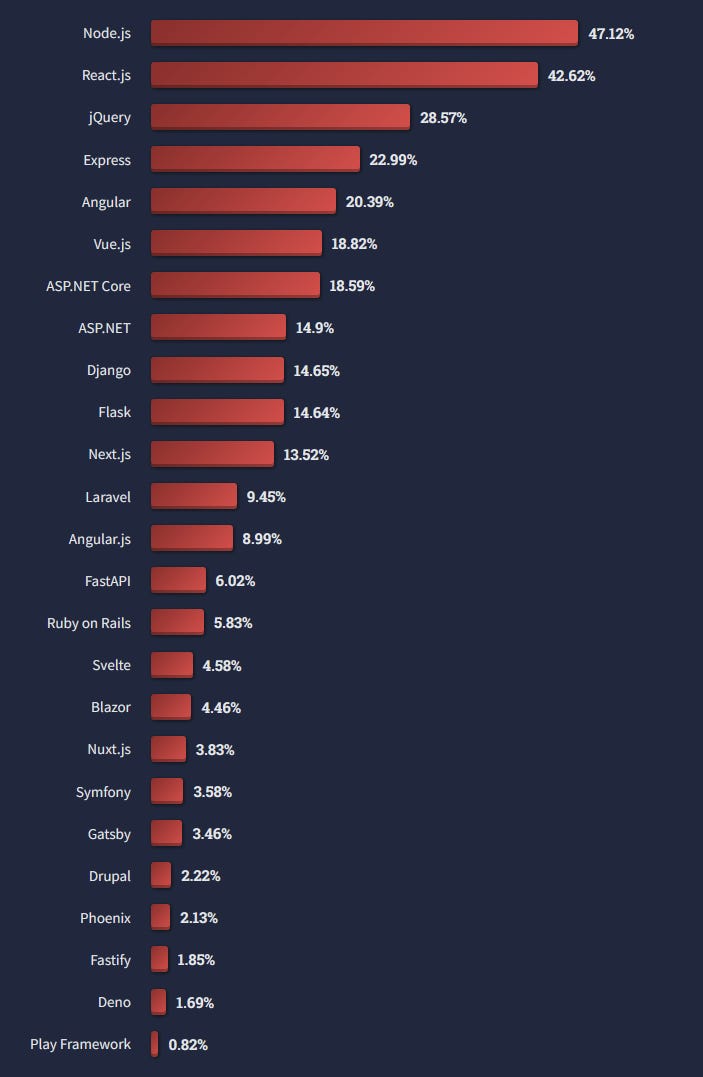
Community numbers
Here we will use numbers from Github and Stack Overflow (If you have a suggestion for a better way to gauge this, let me know in the comments).
The amount of questions on Stack Overflow will give an indication of how easy it is to find answers when you run into problems.
React:
Released 2013
Maintained by Meta
200k stars on Github
431,729 questions tagged with react on Stack Overflow
Vue:
Released 2014
Maintained by Evan You and the rest of the Vue team.
200k stars on Github
100,095 questions tagged with Vue on Stack Overflow
Angular:
Released 2016
Maintained by Google
85k stars on Github
288,323 questions tagged with Angular on Stack Overflow
Svelte:
Released 2016
Maintained by Rich Harris, Simon Holthausen, and others at Vercel.
63k stars on Github
4,027 questions tagged with Svelte on Stack Overflow
jQuery
Released 2006
Maintained by The jQuery team
57k stars on Github
1,031,599 questions tagged with jQuery on Stack Overflow
The amount of jQuery questions on Stack Overflow speaks of its high usage.
Enjoyment
Lets first see what the survey says. The State of JS Survey used a Positive/negative split format, and the Stack Overflow Developer Survey used a Loved vs. Dreaded format:
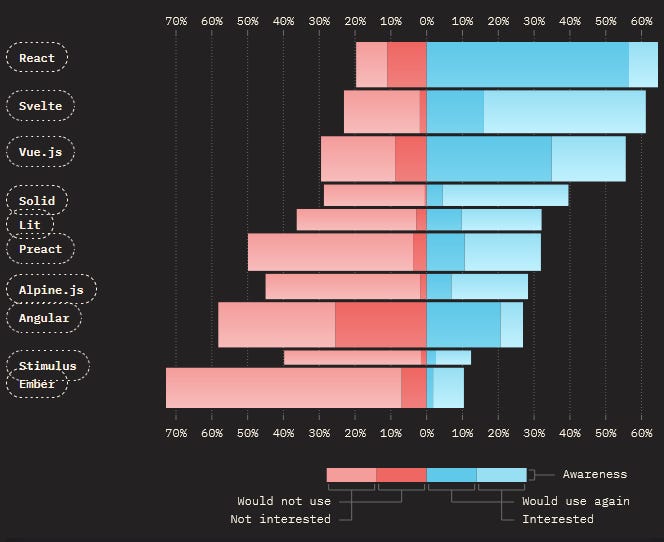
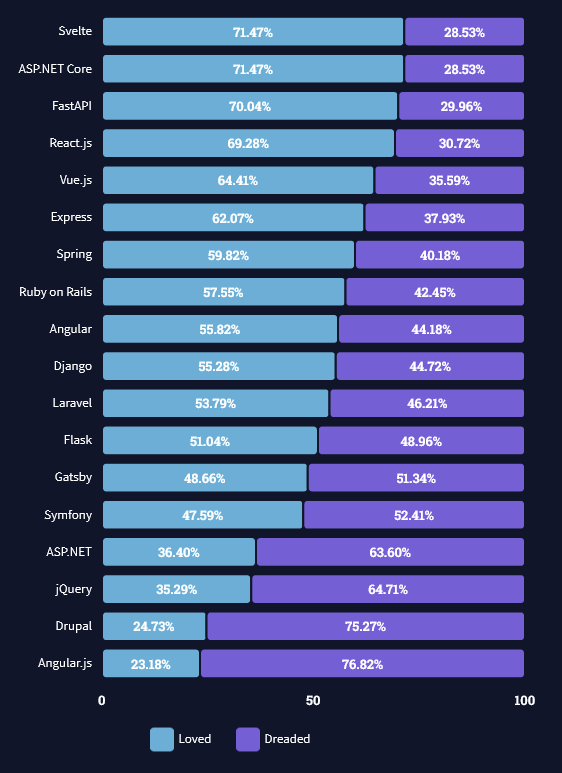
In both of these, React and Svelte comes out at the top, with Vue slightly behind.
In my experience, enjoyment of a web framework comes from simplicity, ease learning and using, and being able to quickly code up a project without jumping through too many hoops or having to google too many things.
I recently learned Svelte and have used it in one project. I liked both how easy it was to understand its way of doing things, and its simple build process and configuration.
I have also used React, and I liked how many resources there was on the web for learning it. For every question I had there was an article, video, or an answer ready on Stack Overflow, or .
I have also used Vue extensively and enjoy its declarative syntax and that it is a progressive framework. There are also many resources on the web for learning Vue.
I am very surprised that jQuery is so dreaded. I find that it is a great library to use when I just want to code something simple that doesn’t need all the firepower that the other modern frameworks has (like reactive states, data store, frontend routing, etc.). Just pop the CDN link for jQuery in the header tag and I’m ready to go!
To sum up. I think all of the ones I mentioned above is great for making UIs. If I was a new web developer with no experience, I would probably go with React due to its big community and vast learning resources available online. Svelte would be my second choice because its simplicity is so nice. And for simple websites I would definitely just use jQuery.
I haven’t tried Angular, so I can’t give any advice on that, but from people I have talked to it seems like individual developers thinks it has too strict rules, while large enterprises likes it because it enforces a certain way of doing things. For enterprises, this makes it easier to maintain large code-bases.
Choosing Frontend stack based on website requirements
In this section we will try to think about how different Frontend web stacks fits different kinds of apps and websites. Starting with a simple static website and building up to a complex modern web app.
We haven’t discussed CSS frameworks. So I am just going to add suggestions for ones I like: TailwindCSS and Bootstrap. I recommend TailwindCSS for most projects. It is a simplistic CSS framework that provides convenience and enjoyment, while not standardizing how the way websites/apps look and feel. Bootstrap is something I use for simple projects where I just want to import a CSS toolkit with a header link and get going.
Basic static site with no or limited interactivity
This is like your local hairdresser type website. These kind of websites are mostly static, with rare updates, and limited amount of UI interactivity.
While something like React would definitely work for this website, it is overkill and would mostly just complicate things. You should only use an advanced framework for this if you already have a good workflow with it.
Suggested stack:
jQuery/Alpine.js + Bootstrap
Just create an HTML5 file, import the jQuery and Bootstrap CDN links, and you are ready to go!
A website with an app-like UI, and no need for SEO
Any website where you expect the user to interact with the site.
Suggested stack:
React + TailwindCSS
Requires a bit of setup, but it is a powerful combination once you get going. Many large and popular websites and apps use React.
Alternatively use Vue, Svelte, or Angular instead of React.
An app-like website, but you have content that needs SEO
This may be any website that uses a frontend web framework like React, but which has content that it wants a search engine crawlers to find. React, Angular, Vue and Svelte are all Single-Page Applications (SPAs), which is bad for SEO, so for this situation we need a Meta/Hybrid framework where Server-Side Rendering can be used.
Suggested stack:
Next.js (React) + TailwindCSS
Or alternatively use Nuxt (for Vue), SvelteKit (for Svelte), or Angular Universal (for Angular).
Conclusion
This post has evaluated frontend frameworks and here is my recommendation:
Overall winner: React and Next.js + TailwindCSS
React is a JavaScript frontend framework for making highly interactive UIs.
TailwindCSS is a utility-first CSS framework for rapid UI development.
Use Next.js (a React meta-framework) if you need server-side rendering (SSR), e.g., for Search-Engine Optimization (SEO).
For websites that needs any sort of interactive UI (which includes most modern websites and apps), React is a good choice. It has been the most used javascript frontend web framework for at least five years and the community is huge.
Alternative for very simple websites: jQuery / Alpine.js + Bootstrap
jQuery is a JavaScript library designed to simplify HTML DOM tree traversal and manipulation, as well as event handling, CSS animation, and Ajax. It has an easy-to-use API that works across a multitude of browsers. Installing jQuery is easy, just add a script tag with a CDN link in your HTML document.
Alpine.js is a minimal tool for composing behavior and interactive UIs directly in your markup. Think of it like jQuery for the modern web. Installing it is as easy as jQuery, just pop in a script tag and get going.
Bootstrap is a CSS toolkit with grids, ready-made components, icons, and more. They have a CDN version that can be included directly into the HTML document.
For very simple websites/apps, React can be a little bit cumbersome. jQuery and alpine.js can be directly imported into the HTML document and you can get going with a very simple setup.
jQuery is still used on the majority of all websites, and used by 29.21% of professional developers (placed after react at 44.31%).
Thanks for reading! Please leave a comment below with feedback. Did you like it? Was it useful? Was there something you wished I would have discussed?
Have a great day,
- Eric